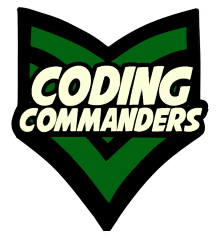
HTML + CSS Side Menu
In this lesson you will learn to make the side navigation menu. In the next lesson we will learn to turn it into a hamberger menu when the user is on a mobile device. This lesson refers to the code on Twitch Website Builder. To see the site live visit Twitch Website Sample.
Run The Code
The desktop navigation menu is created with HTML + CSS. We have a container called "sidenav" containing navigational links (<a>)
HTML
<div class="sidenav" id="sidenav">
<!-- Side Navigation Heading -->
<h3>Menu</h3>
<!-- Side Navigation Links -->
<a href="index.html">Home</a>
<a href="https://www.twitch.tv/daisychaincosplay" target="blank">Twitch Channel</a>
<a href="about.html">About Me</a>
<a href="https://www.codingcommanders.com/website-builder">Twitch Website Builder</a>
<a href="https://www.codingcommanders.com/battlecards">Battle Card RPG</a>
<a href="https://www.codingcommanders.com/godot">Godot Tutorials</a>
<a href="https://www.codingcommanders.com/linux-gaming">Linux Gaming Blog</a>
<a href="https://www.codingcommanders.com/nodejs">NodeJS + Postgres</a>
<a href="https://www.codingcommanders.com/LAMP">LAMP Stack</a>
<a href="https://www.codingcommanders.com/html">HTML</a>
<a href="https://www.codingcommanders.com/css">CSS</a>
<a href="https://www.codingcommanders.com/php">PHP</a>
<a href="https://www.codingcommanders.com/reactVRMain.php">ReactJS VR</a>
<a href="https://www.codingcommanders.com/blog">Tech Blog</a>
<a href="http://www.palmbeachtechie.com/" target="blank">Palm Beach Techie</a>
<a href="https://streamlabs.com/daisychaincosplay/tip" target="blank">Twitch Donations</a>
<a href="http://codingcommanders.storenvy.com/" target="blank">T-Shirt Shop</a>
<a href="https://donorbox.org/18be1a20-283d-414d-bae0-fbf1d7c101db" target="blank">DonorBox</a>
</div>
CSS
/* Side Navigation Container */
.sidenav {
background-color: #111;
padding-top: 60px;
padding-bottom: 60PX;
height: 100%;
border-radius: 9px;
}
/* Side Navigation Links */
.sidenav a {
display: block;
color: #818181;
padding: 8px 8px 8px 32px;
font-size: 26px;
border-radius: 6px;
}
/* When mouse hover on links in side Navigation */
.sidenav a:hover {
background-color: darkred;
color: white;
-webkit-transition: background-color 0.6s ease-out, color 0.6s ease-out;
-moz-transition: background-color 0.6s ease-out, color 0.6s ease-out;
-o-transition: background-color 0.6s ease-out, color 0.6s ease-out;
transition: background-color 0.6s ease-out, color 0.6s ease-out;
}
/* Side Navigation Heading */
.sidenav h3 {
padding: 8px 8px 8px 32px;
}
Video: HTML Images, iframes, Videos, and Navigational Links
Formatting Navigational Links
To learn how to format navigational links, check out: CSS - Style Navigation Links. That tutorial describes the basic link properties and how to cutomize them.
CSS tranitions
Now that you have learned basic link formatting, let's get into something a bit more advanced. Using CSS transitions to slowly change color on hover.
Transitions are a way to gradually change a specific property (i.e. width, color, opacity) when a particular event takes place. In this example, we are changing a link's background-color and text color on hover. We do not want the change to happen instantaneously. We want the color to gradually change over .6 seconds of time.
.sidenav a:hover {
background-color: darkred;
color: white;
transition: background-color 0.6s ease-out, color 0.6s ease-out;
}
The code above will apply to all navigation links inside "sidenav" elements. When a user hovers over a link in the side nav menu, the text color with gradually change to white and the link's background color will gradually change to dark red. You can check it out in the code ediitor box above.
However, we are not done yet! We want to make sure all common web browsers can understand this transition. Some older browsers need a prefix in order to read the code.
.sidenav a:hover {
background-color: darkred;
color: white;
-webkit-transition: background-color 0.6s ease-out, color 0.6s ease-out;
-moz-transition: background-color 0.6s ease-out, color 0.6s ease-out;
-o-transition: background-color 0.6s ease-out, color 0.6s ease-out;
transition: background-color 0.6s ease-out, color 0.6s ease-out;
}
Menu Containers
On our Twitch website, our side menu container is called "sidenav". To learn more about making menu containers check out CSS Menus.