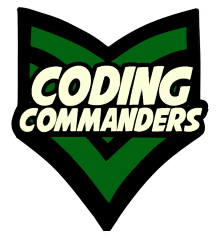
JavaScript Hamburger Menu
Are you ready to start the wondrous journey of JavaScript? In this lesson, we will learn to use JavaScript to create a hamburger menu. On mobile devices, the side menu will turn into a hamburger menu on the page header.
Run The Code
Responsive Design
You may find it helpful to review Coding Commanders Responsive Design Tutorials. In this lesson we will use media queries to display the page differently on mobile devices.
How is the mobile view different?
On devices 768px wide and smaller, we reduce the <h1> size to 32px. We also reduce the <h1> top-margin to 0.
/* Smaller h1 heading on small devices */
@media only screen and (max-width: 768px) {
h1 {font-size: 32px; margin-top: 0px;}
}
When users are on large devices, we do not want them to see the mobile menu.
CSS
.mobile-menu {
visibility: hidden;
}
HTML
<button onclick="mobileMenu" id="menu-link" class="mobile-menu"><i class="fas fa-bars"></i></button>
When on small devices, you want the side menu and side images invisable and we want our mobile menu icon visable. We also change the style of the sidenav so (using JavaScript) we can stick it into our mobile menu.
/* Switch to hamburger menu on small devices */
@media only screen and ( max-width:768px) {
.mobile-menu {visibility: visible;}
#menu {display: none;}
#menu-display {display: block; transition: .6s;}
.sidenav {background-color: rgb(52,26,35,.5); }
}
JavaScript hamburger Menu
index.html
<!-- Mobile hamburger Menu Icon (Mobile Only) -->
<button onclick="mobileMenu" id="menu-link" class="mobile-menu"><i class="fas fa-bars"></i></button>
<!-- Mobile Menu Container -->
<div id="menu-display"></div>
main.js
function mobileMenu() {
var node = document.getElementById("sidenav");
document.getElementById("menu-display").appendChild(node);
toggle();
}
function toggle() {
var x = document.getElementById("menu-display");
if (x.style.display === "block") {
x.style.display = "none";
}
else {
x.style.display = "block";
}
}
document.getElementById("menu-link").addEventListener("click", mobileMenu);
➼ mobileMenu - Our mobile menu function is referenced in the html button element above. When a user clicks on the hamburger menu icon, the code will execute.
The side menu is placed inside the mobile menu container and toggle() is called.
➼ toggle - This function allows the user to toggle between showing and hiding the mobile menu.
➼ Event Listener - Event listeners listen for specific events. In this case it is listening for when the user clicks on the hamburger menu icon.
index.html
<!-- Mobile hamburger Menu Icon (Mobile Only) -->
<button onclick="mobileMenu" id="menu-link" class="mobile-menu"><i class="fas fa-bars"></i></button>
<!-- Mobile Menu Container -->
<div id="menu-display"></div>
mobileMenu function: Create the mobile menu
function mobileMenu() {
var node = document.getElementById("sidenav");
document.getElementById("menu-display").appendChild(node);
toggle();
}
➼ node - node is a variable name. It is set equal to the element with the ID "sidenav".
➼ menu-display - The container we made for the mobile menu has the ID "menu-display". We are taking the contents of node and placing it inside our menu-display element.
➼ toggle() - The code in the toggle function executes.
toogle function: Show hide mobile menu
function toggle() {
var x = document.getElementById("menu-display");
if (x.style.display === "block") {
x.style.display = "none";
}
else {
x.style.display = "block";
}
}
➼ x - x is equal to the element with the id "menu-display".
➼ x.style.display === "block" - If "menu-display" is currently displaying as a block, we want to stop displaying it.
➼ else - In any other case, we want "menu-display" displayed as a block.