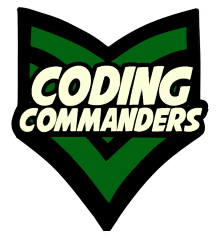
JavaScript Random Number Generator
In this lesson, we build a vanilla JavaScript random number generator. The user enters a minimum and maximum value and our JavaScript will generate a pseudo-random integer.
Why Pseudo-Random?
In this lesson we use the JavaScript pre-defined function Math.random() to generate a pseudo-random number. "Pseudo" means "not genuine" or "a sham".
Math.random uses an algorithim to simulate randomness. Because an algorithim is used, there is no way to produce actual randomness. Random means "chosen without method or conscious decision". The algorithm is the method used. If you know the algorithm, you can use it to predict results.
Don't get caught up on "pseudo". JavaScript, like other major programming languages, does a really good job at simulating randomness. It does so well, you can think of the results as a "random number".
Project File Structure
The file structure is how you organize the files in your project.
Mouse Hover over the ➼ blue text in the description to highlight.random-number-generator
index.html
random.css
chime.mp3
➼ Main Project Folder - All our project files go in here
➼ index.html - This is the main page users will be directed to when entering your application. HTML is the markup language that displays your content in the web browser. Our JavaScript Code is also located in this file.
➼ random.css - CSS formats HTML: color, size, shape, font ect.
➼ sound file - Our JavaScript button makes this sound!
Project Code
Below you will find quick copy boxes filled with the project code.
index.html
*************************
random.css
*************************
Download Sound
Pre-defined JavaScript Functions
Next, I will go ovewr the pre-defined functions we use to generate a random integer.
Math.random()
Math.random() returns a "random" number between 0 and 1. It may return 0, but will always return a number less than 1.
Math.floor()
Math.floor() rounds a number down to the nearest integer.
What will num equal?
var num = Math.floor(Math.random());
num will always equal 0. Why? Math.random() will always return a number less than 1. Then Math.floor will round that number down to 0.
Math.ceil()
Math.ceil() rounds a number up to the nearest integer.
What will num equal?
var num = Math.ceil(Math.random());
num will probably equal 1. In the rare case that Math.random returns 0, it will equal 0.
function randNum(min, max){...}
Now I will go over our JavaScript function used to generate a random integer between min and max.