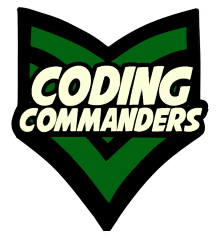
Social Media Links: HTML + CSS
In this lesson, you will learn how to customize and style your own social media links using HTML and CSS. In our Twitch Website Builder Template, the social media links look like this:
Fontawesome
To get the social media icons, I am using the Fontawesome Librbary. To use Fontawesome, you must include the following code in your page head:
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.2.0/css/all.css" integrity="sha384-hWVjflwFxL6sNzntih27bfxkr27PmbbK/iSvJ+a4+0owXq79v+lsFkW54bOGbiDQ" crossorigin="anonymous">
(The Fontawesome link is already included in the template code found on Twitch Website Builder.)
HTML
Social media links are placed inside the "social" container. Each individual link is placed inside link tags. We will use CSS to style our social links.
Mouse hover over the blue ➼ to highlight the code.<!-- Social Links Container -->
<div class="row social" align="center">
<a target = "blank" href = 'https://www.twitch.tv/daisychaincosplay'><i class="fab fa-twitch"></i></a>
<a target = "blank" href = 'https://www.youtube.com/channel/UC-gCUMK_EGUqJ7P9VA7BJmQ'><i class="fab fa-youtube"></i></a>
<a target = "blank" href='https://twitter.com/codingCommander' ><i class="fab fa-twitter"></i></a>
<a target = "blank" href='https://www.facebook.com/codingcommanders/'><i class='fab fa-facebook'></i></a>
<a target = "blank" href='https://www.instagram.com/codingcommanders'><i class='fab fa-instagram'></i></a>
<a target="blank" href="https://social.tchncs.de/@codingcommanders"><i class="fab fa-mastodon"></i></a>
<a target="blank" href="https://discordapp.com/invite/AeUvu7v"> <i class="fab fa-discord"></i></a>
</div>
➼ target - Links with a target attribute open in another tab. The general rule: external links have a target and internal links do not. You want to use the same variable name for each target attribute, so every external link opens on the same tab.
➼ href - The href attribute equals the link to your social media page.
➼ icon - These are the icons that I copied and pasted from Fontawesome.
CSS
Social Container
Our social links are placed inside a social container.
.social {
padding: 15px;
margin: 5px;
border-radius: 6px;
position: relative;
overflow: hidden;
}
➼ padding - CSS Padding Tutorial
➼ margin - CSS Margin Tutorial
➼ border-radius - CSS Border-radius Tutorial
➼ position - CSS Position Tutorial
➼ overflow - CSS Overflow Tutorial
Social Links
The following code styles the links inside our social container. Notice the links display as an inline block. This is usually done with elements you want to place side by side, but also want to set specific height, width, margin, and padding.
/* Social Media Links*/
.social a{
font-size: 30px;
color: #F4FFFE;
border-style: groove;
border-color: grey;
padding: 10px;
margin: 10px;
border-radius: 6px;
display: inline-block
}
Social Links on Hover
The following code describes what happens when a user mouse hovers over the social links.
/* Social Media Links on mouse hover */
.social a:hover {
background-color: darkred;
color: white;
-webkit-transition: background-color 0.6s ease-out, color 0.6s ease-out;
-moz-transition: background-color 0.6s ease-out, color 0.6s ease-out;
-o-transition: background-color 0.6s ease-out, color 0.6s ease-out;
transition: background-color 0.6s ease-out, color 0.6s ease-out;
}
Notice the transitions used. The code is described in the Menus Tutorial.