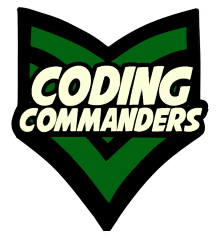
Build a Mobile Hamburger Menu
The devices people use to browse the web vary in size. We often want a website to look different on small devices (i.e., mobile phones) than on larger ones (i.e., computers). We see this concept illustrated by the frequent use of hamburger menus.
In this beginner-friendly tutorial, we will build a responsive mobile hamburger menu using:
✶ HTML
✶ CSS
✶ JavaScript
A regular side menu will display on large devices, and a hamburger menu will display on small ones.
YouTube Tutorial: Mobile Hamburger Menu

What is a Mobile Hamburger Menu?
A hamburger menu is a navigational menu that can be excessed by clicking on an icon with three horizontal lines:
When the user clicks on the icon, the menu gets displayed. When the user clicks on the icon again, the menu disappears. Hamburger menus are handy for mobile devices, that have a smaller display area.