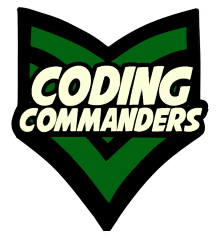
JavaScript If Statements
We use conditional statements to execute code only under certain conditions. Logical operators and comparison operators are used to code these conditions.
If statements are a common type of conditional statement that is directly derived from implication. Learn more about implication in my lesson on Propositional Logic.
Example
You are coding a JavaScript game. If the player scores more points than the enemy, he wins. If the play scores less points than the enemy he looses. If the player and the enemy score the exact same points, it is a tie.
if(player_score === enemy_score){
console.log("It's a tie.");
}
else if (player_score < enemy_score) {
console.log("You Loose!!!");
}
else if (player_score > enemy_score) {
console.log("You Win!!!");
}
else {
console.log("You broke the game!");
}
What will the output be in the following scenarios?
(1)
var player_score = 100;
var enemy_score = 200;
(2)
var player_score = 100;
var enemy_score = 20;
(3)
var player_score = 100;
var enemy_score = 100;
(4)
var player_score = 100;
var enemy_score = "bag";
Answers
- You Loose!!!
- You Win!!!
- It's a tie.
- You broke the game!
Compund Conditions
Sometimes more than one condition must be met for a block of code to execute. We use logical operators to code these conditions. Check out the example below.
Billy is ordering t-shirts. Plain youth shirts cost $10. Graphical youth shirts cost $14. Plain adult shirts cost $15. Graphical adult shirts cost $20. Write the code to output the price of a shirt.