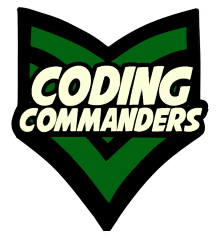
Asynchronous JavaScript Programming
Understanding Asynchronous Programming?
In this article I will explain the difference between synchronous and asynchronous JavaScript.
Synchronous Programming
Synchronous programming executes one line of code at a time. When the code line executes, it requests the result. The application waits for the response. Once the response returns, the next line of code fired off!
Click through the slide below to see the concept illustrated.
Here is an example of synchronous JavaScript code.
// code 1
let num = 8;
// code 2
let triple = num * 3;
// code 3
console.log(triple);
Asynchronous JavaScript Programming
In contrast, asynchronous code does not wait. Code statements will fire off one by one, like with synchronous code. However, the application does not wait for a returned response before moving on to the next code block.
Clcik through the slide below to see the concept illustrated.
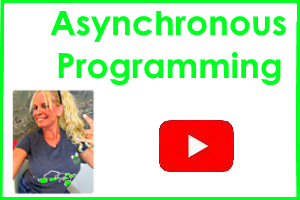
When is Vanilla JavaScript Asynchronous?
JavaScript code is usually asynchronous anytime you have to leave front-end JavaScript for a result. Common asynchronous tasks include:
- Ajax Requests
- Reading/Writing Files
- Timer Functions
Asynchronous code requieres a callback. to handle the results. A call back is a function that is passed into another function. If you pass a callback into an asynchronous function, that callback will wait for a response from the callback.
Asynchronous JavaScript Code
>What is the Benefit of Asynchronous Code?
It doesn't wait. Some code requests (reading a file, calling to the backend server, etc) take longer than valuating a regular JavaScript expression. For these longer requests, we only want the rest of the code to wait if it has to.
For example, let us say you are writing code to create a blog. All the page is created with regular JavaScript expressions, except the article. You have to read the article from an external file. You do not want the rest of the page to wait on that article. The page can create while your file read function waits for a response.