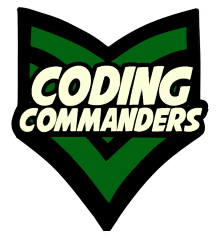
JavaScript Operators
In this lesson you will learn about JavaScript mathematical, logical, comparison operators, and assignment operators.
Mathematical Operators
Operator Name | Symbol | Example |
---|---|---|
Addition | + | var total = price + tax; |
Subtraction | - | var difference = score1 - score2; |
Multiplication | * | var stream_time = time * multiple; |
Division | / | var payout = loot / players; |
Exponent | ** | var area = 10 ** 2; |
Remainder | % | var leftovers = 10 % 3; |
Increment | ++ | score++; |
Decrement | -- | lives--; |
You are probably familar with addition, subtraction, multiplication, and division, so I will not go over those. They work the same in JavaScript as they did in your grammar school math class.
Exponent
A number's exponent states how many time the number will be multiplied by itself.
var side = 6;
var area = side ** 2;
// area is equal to 36 (6*6)
var num = 3 ** 3;
// num is equal to 27 (3*3*3)
Remainder
The remainder is the amount left over after two numbers are divided. Let's say you have a pizza party. You ordered a total of 32 slices of pizza. Ten guests show up. You give each guest the same amount of pizza. How many slices are left?
var leftovers = 32 % 10;
// leftovers is equal to 2
Increment
Increment will add one to the value of any variable. There are two ways to increment: pre-increment and post-increment. Pre-increment is added before the variable name and post is added after the variable name. If you pre-increment one is added before the value is returned. If you post increment the value is returned then one is added. See the code below.
var score = 10;
console.log(score++);
// returns 10
console.log(score);
// returns 11
console.log(++score);
// returns 12
Decrement
Decrement works just like increment, except you subtract 1.
var score = 10;
console.log(score--);
// returns 10
console.log(score);
// returns 9
console.log(--score);
// returns 8
Comparison Operators
Comparison operators are used to compare values. We will use these operators more in my if statements lesson.
Operator Name | Symbol | Example |
---|---|---|
Equal To | == | price == budget |
Equal Value and Type | === | height === width |
Less Than | < | height < width |
Greater Than | > | width > height |
Less Than or Equal To | <= | price &t;= budget |
Great Than or Equal To | >= | budget >= price |
Not Equal To | != | length != width |
Not Equal Value or Type/td> | !== | name !== age |
Logical operators
Logical operators are used in conditional statements such as if statements and switch statements. You will learn more about them in those lessons.
Operator Name | Symbol | Example |
---|---|---|
And | && / and | apples && oranges |
Or | || / or | apples || oranges |
Not | ! | !apples |
Assignment Operators
Symbol | Example | Example Meaning |
---|---|---|
+= | score += bonus | score = score + bonus |
-= | score-=mistakes | score = score - mistakes |
*= | score *= multiplier | score = score * multiplier |
/= | loot /= party_members | loot = loot / party_members |