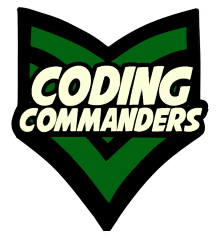
JavaScript Variable Scope and Reassignment
In this lesson, we will discuss the different ways you can declare JavaScript variables and constants relative to variable scope and reassignment.
Variable Scope
A variable's scope refers to the parts of a program where that variable can be accessed. To determine how and where to declare your variable, you must understand the different variable scopes.
Global Variables
Global Variables are declared outside of any functions. These variables can be accessed and manipulated both inside and outside functions.
// global variable
var username = "LinuxKing";
function sayMyName() {
console.log(username);
}
sayMyName();
Local Variables
Local variables are declared inside a function. They only exist inside that function. They can not be accessed or altered outside of the function.
function sayMyName() {
// local variable
var name = "Sam";
console.log(name);
}
sayMyName();
Function Scope
Variables declared with function scope are only accessable inside that function. The keyword var is used to declare variables within a function.
function getPotatos(){
// function scope variable
var num_potatos = 3;
console.log (num_potatos);
}
If you try to reference a variable outside of its scope, you will get an error.
function getPotatos(){
// function scope variable
var num_potatos = 3;
}
console.log(num_potatos);
Output:
But, if we declare a var variable inside a code block, such as a conditional statement or a loop, we can access it throughout code.
var num_carrots = 8;
if(num_carrots > 3) {
var happiness = 10;
}
console.log(happiness);
Output:
Block Scope
A block of code is incased inside {}. This includes functions, loops, and conditional statement. Variables declared with the keyword let or the keyword const have block scope. That means they only exist inside the code block in which they are declared.
var num_carrots = 8;
if(num_carrots > 3) {
let happiness = 10;
}
console.log(happiness);
Output:
Value Reasignment
Variables are declared with var or let and their values can be reasigned.
const FL_SALES_TAX = .06;
let shopping_bill = 100;
if (shopping_bill < 150) {
shopping_bill += 25;
}
console.log(shopping_bill);
Output:
Constants are declared using const and are usually named in all capital letters. A constant's value can not be reassigned.
const FL_SALES_TAX = .06;
let shopping_bill = 100;
if (shopping_bill < 150) {
FL_SALES_TAX += .02;
}
console.log(FL_SALES_TAX);