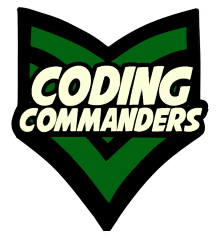
Introduction to JavaScript Variables
Variables store data values. Each variable has a name, called an identifier. A variable's name is used to identify it's stored value.
Naming Variables
Identifiers must start with a letter and may contain:
- Letters
- Numbers
- Underscores
- Dollarsigns
They are case sensitive, so "myVariable" would point to a different value than "myvariable". JavaScript keywords can not be used as variable names.
Data Types
Each variable has a data type. This refers to the type of data the variable stores. Here is a list of JavaScript datatypes:
String
A string variable stores characters. The value is placed in quotation marks. See the example below.
var myStringvariable = "Hi, I am a string!";
var username = "cylon6";
Number
Number variables can store whole nubers or decimals.
var salesTax = .06;
var count = 36;
Boolean
Boolean variables have 2 possible values: true or false.
permission1 = true;
permission2 = false;
Reviewing propositional logic will help you understand boolean variables.
Array
An array stores a set of data. You will find more information on arrays in JavaScript Datasets
var games = ["Dungeons & Dragons", "Spades", "Twister"];
var grades = [96, 88, 75, 63, 58];
Object
An Object also stores sets of data. This data includes variables called properties and functions called methods. Learn more about objects in JavaScript Datasets and Objects and Constructors.
var user = {username: "cylon6", password:"jsakhd&8hj*hHG5", email: "byyourcommand@basestar.com"};
Null
A null variable does not yet have a value. You can declare a variable as null then later give it a value.
var average = null;
Undefined
An undefined variable has a value that is unkown or does not exist. If you reference a variable that isn't initalize or an object property that does not exist you will get "undefined". If you reference a variable before it has been assigned a value you will also get "undefined".
Loose Typing
JavaScript is a loosely typed language. When you declare a JavaScript variable, you do not have to specify its data type. A JavaScript variable receives its type from the value you store inside it. So a variable's type can change during the execution of a JavaScript application.